SvelteKit 1—Getting Started with SvelteKit
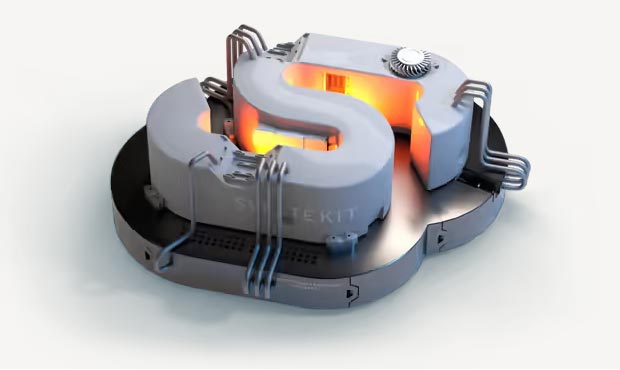
This post will give you a basic introduction to SvelteKit and walk you through building a basic SvelteKit website. If you already know how to do this, you can probably skip this post and go to one of the subsequent ones. Alternately, you can go to kit.svelte.dev both to learn more about what SvelteKit does and how to use it.
What Is SvelteKit?
According to their website, “SvelteKit is a framework for building web applications of all sizes, with a beautiful development experience and flexible filesystem-based routing.
“Unlike single-page apps, SvelteKit doesn’t compromise on SEO, progressive enhancement or the initial load experience — but unlike traditional server-rendered apps, navigation is instantaneous for that app-like feel.”
Let’s Learn by Doing!
In order to go forward, you will need at least a basic familiarity with using the command line. You will also need to have npm installed. To check, enter npm -v at the command line. If you have it, you will get a version number in response. If you don’t already have it, instructions for downloading and installing it are found here.
So create a directory where you will install it:
mkdir my-app && cd my-app
Then run the following command:
npm create svelte@latest .
This will ask you a series of questions. We’ll start off with these answers:
Where should we create your project? (Return).
Which Svelte app template? — (Skeleton project)
Then it will ask if you want to use TypeScript, ESlint, Prettier, and Playwright.
For now, we won’t use TypeScript (choose None), and you can reply No to the
other three questions as well.
Next, enter npm install and wait for a moment while needed software is installed.
Then you can enter
npm run dev -- --open
and your skeleton app will open in
a new browser window, at localhost:5173. (A different port may be specified).
It doesn’t do much yet—just says “Welcome to SvelteKit.”
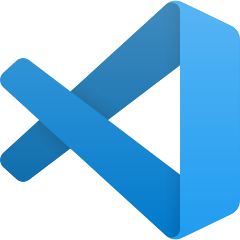
Now, of course, you will want to explore and edit the files that have been created. There are many editors that have settings for Svelte available, and if you have a favorite, feel free to use it. But the most popular with Svelte users is VS Code.
You will want to install the extension “Svelte for VS Code” and perhaps “Svelte Intellisense.” It also helps to go to settings, Text Editor, Suggestions, and disable Accept Suggestion on Commit Character.
So take a look at the directory structure that has been created for you. At the top level are a configuration file, a readme, and a couple of .json files. A helpful .gitignore file is in place for users of git. The node_modules directory contains a suprisingly large number of packages that can be used by your app. Ignoring the .svelte-kit directory, the two remaining directories are where you will do all your work: src and static.
The static directory holds static assets such as image files or global CSS files that can be included in your app. They can be referenced as if at the top level of your app—for example, an img.jpg file contained there can be referenced simply as “/img.jpg”.
The src directory is where you will do essentially all your coding. Initially, it contains an app.html file, which holds the basic html code for your app (but which you mostly won’t need to modify), and a routes directly.
Currently, the routes directory contains just one file, +page.svelte. This is the home page
of our app. All pages are required to have this rather odd name.
Open it in your editor and you will see the source code of the contents
displayed in your browser. Try adding a third line of code, say
<h2>It’s going to be great!</h2>
and, with the browser window showing, hit Save in your editor. Bingo! In a flash, your
browser window updates to show your updated code. This hot updating is one of the
features that makes coding in SvelteKit a pleasure.
Let’s add an About page. The route “about” is produced simply by creating an about subdirectory and adding an +page.svelte file there. Do that, and enter the following code in your new page file:
<h1>About My App</h1>
<p>This is a work in progress.</p>
Save, and then navigate in your browser to localhost:5173/about and you will see your new page.
Web pages typically have a header, a footer, and some common CSS. We can add that to our pages by creating a new file in our routes directory with the special name +layout.svelte. Create that, and add the following code:
<nav>
<a href="/">Home</a>
<a href="/about">About</a>
</nav>
<main>
<slot />
</main>
<style >
:global(body) {
margin: 1em 3em;
background-color: antiquewhite;
}
nav {
font-size: 1.5em;
padding: 1em;
background-color: tan;
}
nav a {
padding-right: 3em;
}
</style>
When you save this, you will see a sudden transformation of your page. You now have a simple menu that lets you move between your two pages, and a background color.
Looking at the code we added, we see several items of interest. First, there is the <slot /> tag. In general, Svelte uses slots as a placeholder for children of a component. In this case, our pages are treated like children of the +layout.svelte component.
Next, we see that the <style> section follows the HTML code. There are three basic sections in a .svelte file—a script section, an HTML section, and a style section. These can be placed in any order, but the tutorials use the order just listed.
Third, the first line in the style section is
:global(body) {
Styles in a .svelte file are scoped to that file. This eliminates the many problems
found in CSS files that can run to thousands of lines, that can lead to hard-to-find bugs
in styling. If you have a lot of global styles, they can be put in a stylesheet linked
in the head of the HTML file (either in app.html or in your +layout.svelte file in a
<svelte:head> section.
However, a style can be made global by embedding it in :global().
Another remarkable feature of styles in Svelte is that unused styles are pruned away, and a warning message is given. This is great when bringing in a huge stylesheet (like BootStrap) where you may be using just a few of the styles. In this case, putting in body without the :global wrapper gave me a warning message saying that body was unused, and so the style was not applied. That alerted me that I needed it to be global so that it would be applied.
SvelteKit can use your favorite preprocessors with no problem—TypeScript, CoffeScript, Markdown, Pug, Less, Sass, PostCSS, Stylus, and more. Details will be given in my upcoming post on how I built this blog.
Svelte is used to create reusable components. Let’s add a simple Footer component to our nascent app. Create a directory under src called components. There’s nothing special about that name; you could call it whatever you like. Create a file in that directory called Footer.svelte, and add the following code:
<script>
export let author;
</script>
<footer>
<p>Copyright © { new Date().getFullYear() }
{#if (author > "")}
by { author }
{/if}
</p>
</footer>
<style>
p {
color: antiquewhite;
}
footer {
background-color: darkblue;
padding: 2em;
text-align: center;
}
</style>
The export statement is Svelte’s way of identifying that a variable is a property, or prop, that is to be passed to the component. On the line with the word Copyright, we see that we can include arbitrary JavaScript in our HTML within curly braces, and it will be evaluated when the page is rendered. The next lines show that chunks of HTML can be included or excluded based on logic. (There is also an #else statement).
Note that in the style section, we have set the color for p elements in the Footer as antiquewhite. Because styles are scoped, this cannot bleed into other areas of our app but applies only to p elements in this component.
Now return to +layout.svelte, and we’ll import and use this component. Add the following three lines to the beginning of the file:
<script>
import Footer from '../components/Footer.svelte';
</script>
Then, just below the </main> line, add the following line of code (replacing my name with yours):
<Footer author="Wahhab Baldwin" />
Voila! We now have a colorful footer on each of our pages. If you replace the Footer invocation with just a plain <Footer /> statement, you will see that the word “by" goes away on the footer because of the #if block.
Congratulations! You’ve created a basic SvelteKit app. Of course, we’ve just scratched the surface of all there is to SvelteKit. The next post will pick up from here and go a bit deeper, learning about getting data from endpoints. See you there!